728x90
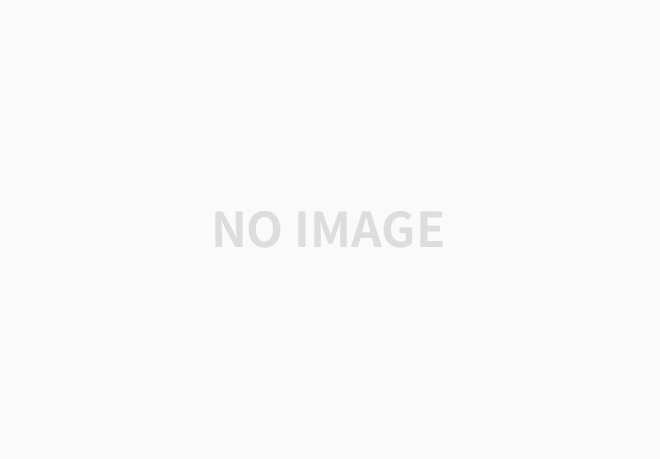
네이밍서버 (유레카 서버)
여러 포트 번호를 쓰고 있기 때문에
네이밍 서버를 통해 포트 번호에 상관없이 가져옴.
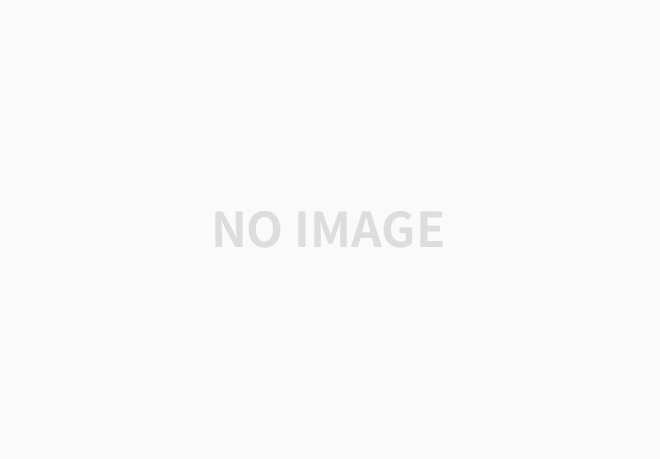
Eureka 유레카를 통해 관리
네이밍 서버
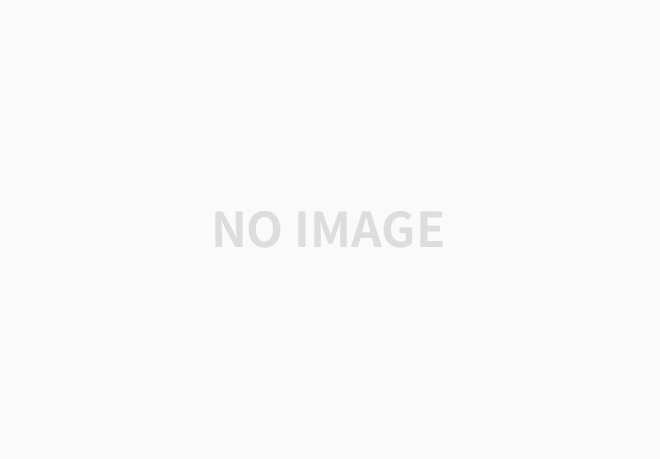
naming server 생성
의존성 등록(maven)
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
Gradle
plugins {
id 'java'
id 'org.springframework.boot' version '2.7.6'
id 'io.spring.dependency-management' version '1.0.15.RELEASE'
}
group = 'com.javapp'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '11'
configurations {
compileOnly {
extendsFrom annotationProcessor
}
}
repositories {
mavenCentral()
}
ext {
set('springCloudVersion', "2021.0.5")
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter'
implementation 'org.springframework.cloud:spring-cloud-starter-netflix-eureka-server'
compileOnly 'org.projectlombok:lombok'
developmentOnly 'org.springframework.boot:spring-boot-devtools'
annotationProcessor 'org.projectlombok:lombok'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
dependencyManagement {
imports {
mavenBom "org.springframework.cloud:spring-cloud-dependencies:${springCloudVersion}"
}
}
tasks.named('test') {
useJUnitPlatform()
}
앱 설정
spring.application.name=naming-server
server.port=8761
eureka.client.register-with-eureka=false
eureka.client.fetch-registry=false
메인
@EnableEurekaServer
@SpringBootApplication
public class NamingServerApplication {
public static void main(String[] args) {
SpringApplication.run(NamingServerApplication.class, args);
}
}
currency-conversion , currency-exchange 마이크로 서비스
의존성 등록(maven)
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
(Gradle)
plugins {
id 'java'
id 'org.springframework.boot' version '2.7.6'
id 'io.spring.dependency-management' version '1.0.15.RELEASE'
}
group = 'com.javapp'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '11'
configurations {
compileOnly {
extendsFrom annotationProcessor
}
}
repositories {
mavenCentral()
}
ext {
set('springCloudVersion', "2021.0.5")
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.springframework.cloud:spring-cloud-starter-netflix-eureka-client'
implementation 'org.springframework.cloud:spring-cloud-starter-openfeign'
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
implementation 'mysql:mysql-connector-java'
implementation 'org.springframework.boot:spring-boot-starter-security'
implementation 'org.springframework.security:spring-security-test'
runtimeOnly group: 'io.jsonwebtoken', name: 'jjwt-jackson', version: '0.11.5'
implementation group: 'io.jsonwebtoken', name: 'jjwt-impl' , version: '0.11.5'
implementation group: 'io.jsonwebtoken', name: 'jjwt-api', version: '0.11.5'
implementation 'org.springframework.boot:spring-boot-starter-validation'
compileOnly 'org.projectlombok:lombok'
developmentOnly 'org.springframework.boot:spring-boot-devtools'
annotationProcessor 'org.projectlombok:lombok'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
dependencyManagement {
imports {
mavenBom "org.springframework.cloud:spring-cloud-dependencies:${springCloudVersion}"
}
}
tasks.named('test') {
useJUnitPlatform()
}
# 유레카 네이밍 서버 등록
eureka.client.serviceUrl.defaultZone=http://localhost:8761/eureka
Feign 과 Eureka를 사용한 로드밸런싱
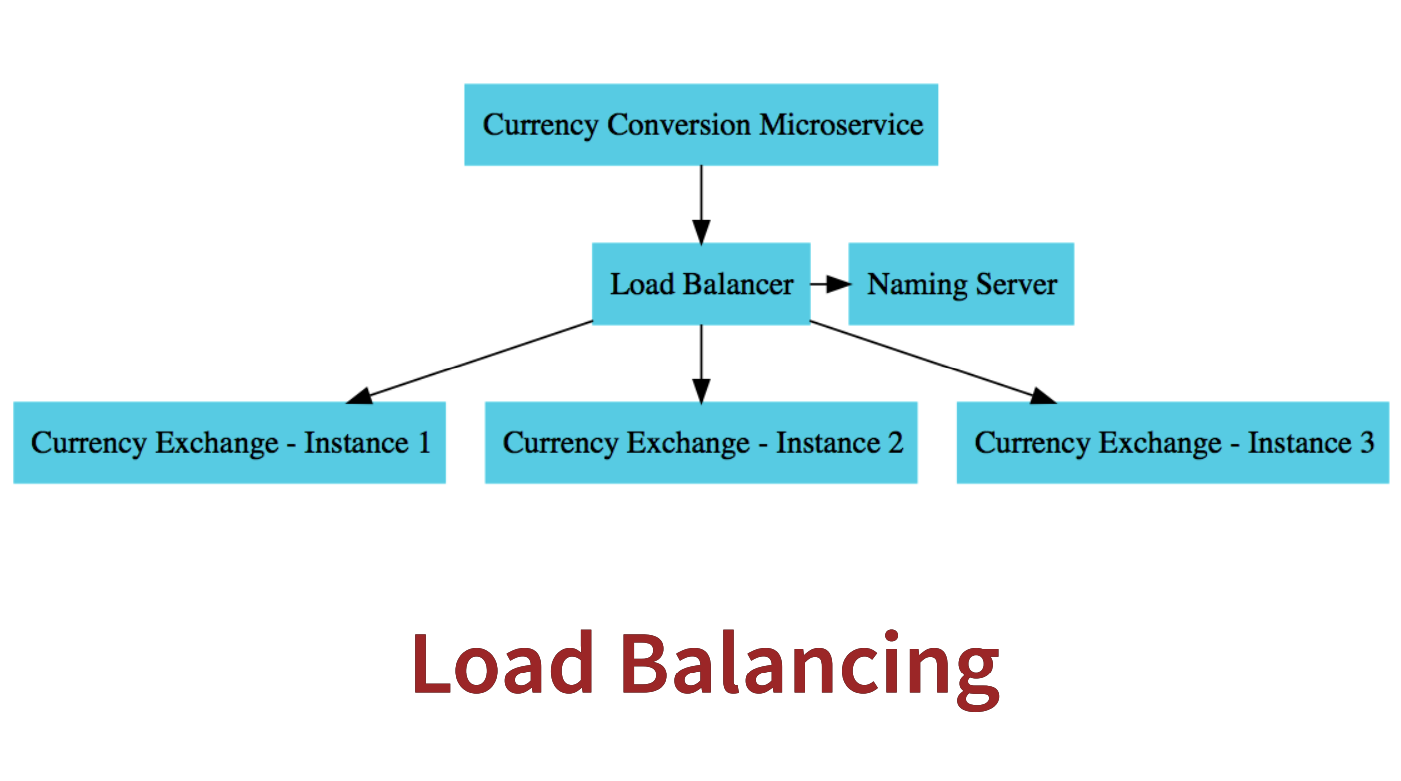
네이밍 서버를 로드밸런싱을 해서 부하를 낮출 수 있음
Currency Exchange 인스턴스 2개 생성

Currency Conversion Microservice
CurrencyConversionController.java
@GetMapping("/currency-converter-feign/from/{from}/to/{to}/quantity/{quantity}")
public CurrencyConversion calculateCurrencyConversionFeign(
@PathVariable String from, @PathVariable String to, @PathVariable BigDecimal quantity
){
CurrencyConversion currencyConversion = currencyExchangeProxy.retrieveExchangeValue(from,to);
return new CurrencyConversion(currencyConversion.getId(),from,to,quantity,
currencyConversion.getConversionMultiple(), quantity.multiply(currencyConversion.getConversionMultiple()) ,currencyConversion.getEnvironment());
}
CurrencyExchangeProxy.java
//@FeignClient(name = "currency-exchange", url="localhost:8000") // properties의 name
@FeignClient(name = "currency-exchange") // properties의 name
public interface CurrencyExchangeProxy
{
@GetMapping("/currency-exchange/from/{from}/to/{to}")
public CurrencyConversion retrieveExchangeValue(
@PathVariable String from, @PathVariable String to
);
}
Currency Exchange Microservice 사용하는 프록시
유레카 로드밸런서 사용
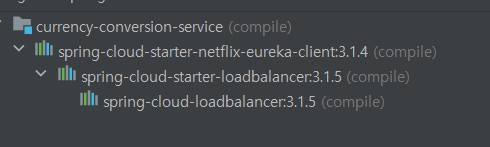
localhost:8100/currency-conversion/from/USD/to/INR/quantity/10

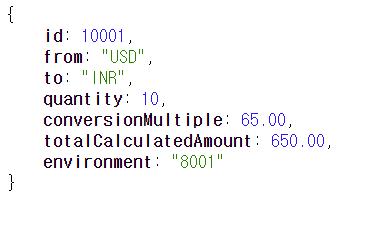
It's automatically load balancing
'Back-end > Spring Cloud (MSA)' 카테고리의 다른 글
Spring Cloud (MSA) - Resilience4j @Retry @CircuitBreaker(서킷브레이커) (0) | 2022.12.06 |
---|---|
Spring Cloud (MSA) API Gateway - 경로탐색(Exploring Routes), Logging Filter (0) | 2022.12.03 |
Spring Cloud (MSA) - Feign 다른 서버 값 가져오기 (0) | 2022.11.26 |
Spring Cloud (MSA) - Config Server (0) | 2022.11.25 |
Spring Cloud (MSA) - HATEOAS, HAL Explorer, Static Filtering, Actuator (0) | 2022.11.24 |
댓글